Imagine you’re scraping a website to extract crucial data, and just as you run your script - bam! - you hit a wall of blocked requests. That’s where BeautifulSoup parse HTML techniques come in, helping you navigate and structure web content effortlessly. But if the site has restrictions, you might need tools like proxies or VPNs to stay undetected.
A proxy acts as an intermediary, rerouting your connection through another server to mask your identity. A VPN (Virtual Private Network), on the other hand, encrypts your internet traffic while also changing your IP address. People use these tools for privacy, bypassing regional blocks, or keeping their web activities hidden. When parsing HTML with BeautifulSoup, knowing how to work around restrictions using proxies can make all the difference.
Now, let’s dive into how BeautifulSoup can help you extract and organize web data like a pro!
What is BeautifulSoup?
BeautifulSoup is a powerful Python library designed to help you extract and manipulate web data from an HTML document or XML file. When dealing with web scraping, raw webpage code can be cluttered with multiple HTML elements, scripts, and unnecessary data. Instead of sifting through the entire page manually, BeautifulSoup allows you to efficiently locate, filter, and organize the information you need.
How Does BeautifulSoup Work?
Webpages are built using HTML tags, which define the structure of a site - like headings, paragraphs, links, and images. Sometimes, the data you need is buried under several layers of nested tags. BeautifulSoup helps by letting you access all the tags in a document or target a specific HTML tag to extract only the relevant content. It also supports working with dynamic web pages, which frequently update their content.
For instance, if you want to extract product prices from an online store or retrieve headlines from a news site, BeautifulSoup makes it easy to parse web data without dealing with the complexities of raw HTML. It even works with wap pages, which are simplified mobile-friendly versions of websites.
Getting Started with BeautifulSoup
To use BeautifulSoup, you first need to fetch a webpage’s content. This is usually done by using the import requests library, which allows you to send a request and retrieve the page’s HTML. Once you have the raw HTML, you create a BeautifulSoup object, which enables you to navigate and extract specific elements effortlessly.
However, some websites restrict or block scraping attempts, especially for dynamic web pages. In such cases, using a residential proxy can help you reach the data without being flagged. You can learn more about them here. Additionally, if you're wondering how web scraping differs from web crawling, this detailed comparison breaks it down.
Image Requirements: Screenshot of BeautifulSoup documentation homepage or a very basic example of HTML being parsed.
Why use BeautifulSoup for parsing HTML?
When it comes to parsing web data, BeautifulSoup is one of the most beginner-friendly and efficient libraries available. Whether you're working with a structured HTML file or a cluttered HTML document, this library makes data extraction simple and intuitive.
Key Benefits of BeautifulSoup
- Easy to Use: Unlike other parsing methods that require complex configurations, BeautifulSoup provides a straightforward way to navigate an HTML document. With just a few lines of code, you can create a soup object and extract relevant HTML data effortlessly.
- Handles Messy HTML: Many websites contain poorly formatted HTML tables, missing tags, or deeply nested elements. BeautifulSoup automatically builds a parse tree, allowing you to extract content even from broken or inconsistent markup.
- Flexible Parsing Options: BeautifulSoup supports multiple parsers, including its built-in html parser and faster alternatives like lxml. You can also use CSS selectors to locate specific elements efficiently.
- Seamless Integration with Requests: Typically, you start by using import requests to fetch webpage content and then use soup beautifulsoup to parse it. This combination makes it incredibly easy to scrape and analyze websites.
How Does It Compare to Other Tools?
While lxml is faster and better suited for large-scale parsing, BeautifulSoup is more forgiving with messy markup. Using regex for web scraping is highly unreliable, as it lacks the structured approach of a proper HTML parser. In most cases, BeautifulSoup strikes the right balance between ease of use and functionality.
Handling Web Scraping Challenges
Even with a great tool like BeautifulSoup, web scraping isn’t always smooth. Websites may block requests, implement CAPTCHAs, or frequently change their structure. To tackle these web scraping challenges, check out this in-depth guide. Additionally, if you're looking for other data extraction tools, this list of top web scraping tools can help you explore more options.
How to install BeautifulSoup
Before you can start web scraping, you need to install BeautifulSoup, a powerful Python library designed to help you parse and navigate HTML documents, XML files, and other web content. Let’s go step by step to get BeautifulSoup up and running.
1. Install BeautifulSoup4 with pip
To install BeautifulSoup4, simply run the following command:

This installs the latest version of BeautifulSoup, which is compatible with Python 3.x. If you haven’t installed Python yet, make sure you have Python 3.6 or higher, as older versions may not support all features of BeautifulSoup.
2. Install an HTML Parser
BeautifulSoup needs a parser to read and process the HTML structure of web pages. By default, Python comes with the built-in html.parser, but you can install a faster alternative like lxml for better performance.
To install lxml, use:

Or, if you prefer the html5lib parser (useful for handling modern HTML5 documents), install it with:

Once installed, you can choose the parser when creating a BeautifulSoup object:

3. Verify the Installation
To confirm that BeautifulSoup and the required parser are installed, open a Python shell and run:

If you see no errors, everything is set up correctly!
4. Why Do You Need BeautifulSoup?
Now that you’ve installed BeautifulSoup, you can start using it to extract data from HTML documents, including text, links, images, and even structured HTML tables. BeautifulSoup makes it easy to locate specific HTML tags, access HTML elements, and filter out unnecessary data.
If you’re working with a web scraping API, using BeautifulSoup alongside requests can help you fetch and process data from different web pages effortlessly. You can also extract all the tags in a document or focus on specific sections of an HTML file.
Basic usage and setup
Now that you have BeautifulSoup installed, let's dive into how to use it for parsing an HTML file. Whether you're working with a saved webpage or scraping live HTML data, BeautifulSoup makes it easy to navigate and extract the content you need.
1. Creating a Simple HTML Document
To get started, let’s create a small HTML file with some basic content. We'll use this sample HTML to demonstrate how BeautifulSoup can find and extract specific elements.

2. Parsing HTML with BeautifulSoup
Now, let’s write a Python script to parse this HTML data and extract specific elements using BeautifulSoup.
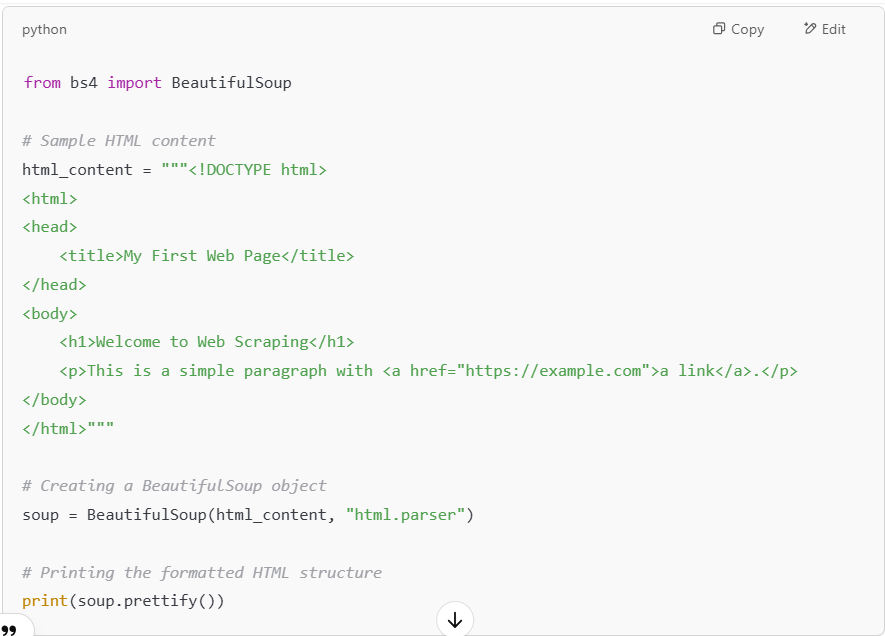
What Does soup = BeautifulSoup(html_content, "html.parser") Mean?
- html_content contains the raw HTML data (our webpage content).
- BeautifulSoup(html_content, "html.parser") creates a BeautifulSoup object, which lets us interact with the HTML file like a structured document.
- The "html.parser" tells BeautifulSoup to use Python’s built-in HTML parser, though you could also use "lxml" for faster processing.
- soup.prettify() prints a nicely formatted version of the HTML, making it easier to read.
3. Extracting Specific Elements
Once we have the BeautifulSoup object, we can extract specific HTML data such as titles, headers, paragraphs, and links.
Extracting the Title of the Webpage

- soup.title finds the <title> tag.
- .text extracts just the text inside the tag.
Extracting the Heading (<h1>)

- soup.h1 automatically finds the first <h1> tag in the document.
Extracting the Paragraph (<p>)

- soup.p selects the first <p> tag and extracts the text inside it.
Extracting the Link (<a> tag)

- soup.a finds the first <a> tag.
- ["href"] extracts the link inside the tag.
4. Extracting All Tags
If you need to find all the tags of a certain type, use the .find_all() method.
Extracting All Links

Extracting All Paragraphs

Summary
- BeautifulSoup helps us parse an HTML file and extract useful HTML data easily.
- We create a BeautifulSoup object using soup = BeautifulSoup(html, "html.parser").
- We can extract HTML elements like titles, headings, paragraphs, and links using simple commands.
- The .find_all() method allows us to get all the tags of a certain type.
Now that you understand the basics of using Beautiful Soup, let’s explore how to navigate and search through more complex HTML structures efficiently!
How to find elements with BeautifulSoup
Once you’ve loaded an HTML document into BeautifulSoup, the next step is locating the elements you need. Whether you’re working with web scraping, analyzing web pages, or extracting data for a CSV file, BeautifulSoup provides several methods to find and filter content.
1. Using .find() to Locate a Single Element
The .find() method searches for the first occurrence of a specific HTML tag in an HTML file.
Example: Finding a <div> with an ID
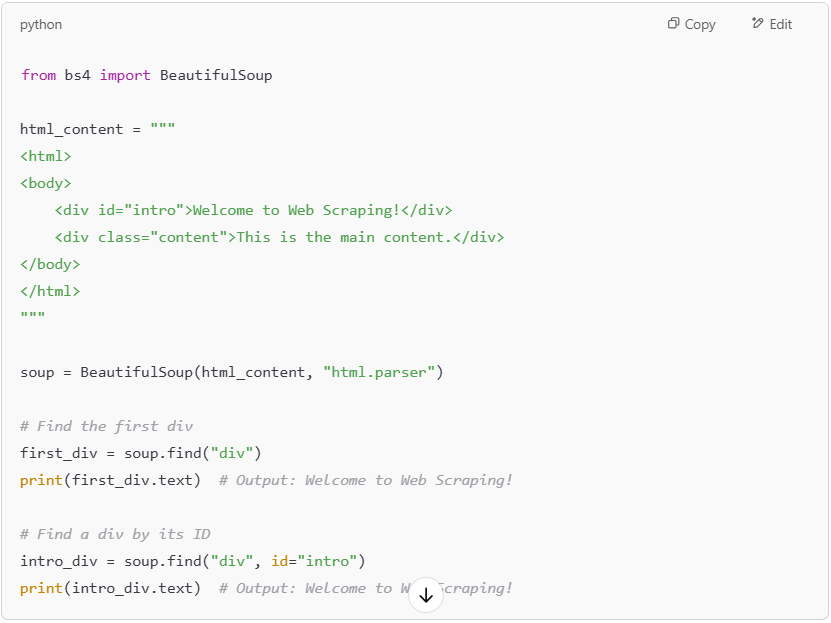
- soup.find("div") finds the first <div> in the HTML document.
- soup.find("div", id="intro") searches for a <div> with id="intro".
2. Using .find_all() to Locate Multiple Elements
If you need to find all occurrences of an HTML tag, use .find_all(). This is useful when working with lists, HTML tables, or multiple paragraphs.
Example: Extracting All Paragraphs
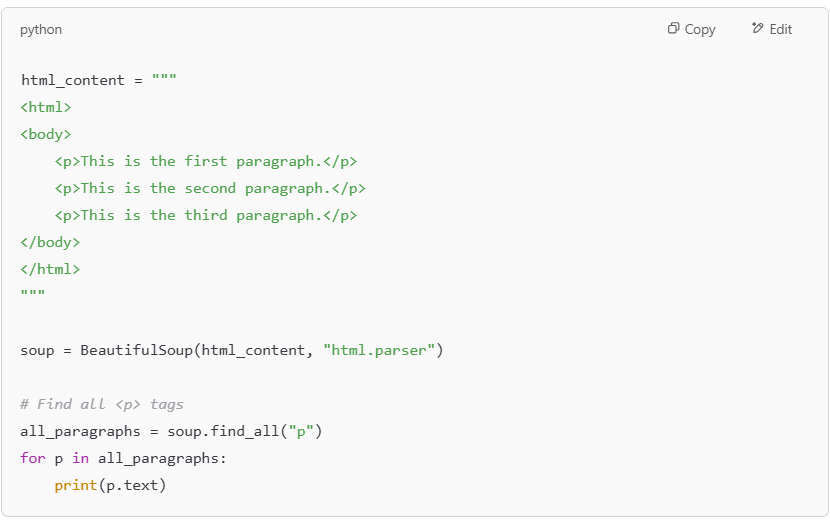
Output:

- .find_all("p") retrieves a list of all <p> tags.
- You can loop through the list to extract and process the text.
3. Finding Elements by Class
If the HTML file has multiple elements with different classes, you can filter them using the class_ parameter.
Example: Finding a <div> by Class

- class_="content" finds the HTML tag with the specified class name.
Finding Elements by ID
Each HTML document can contain elements with unique IDs, making it easier to locate specific sections.
Example: Finding a <section> by ID

Using CSS Selectors with .select()
For more advanced searches, you can use CSS selectors with .select(). This is especially useful when dealing with nested structures in an HTML document.
Example: Using CSS Selectors to Find Elements
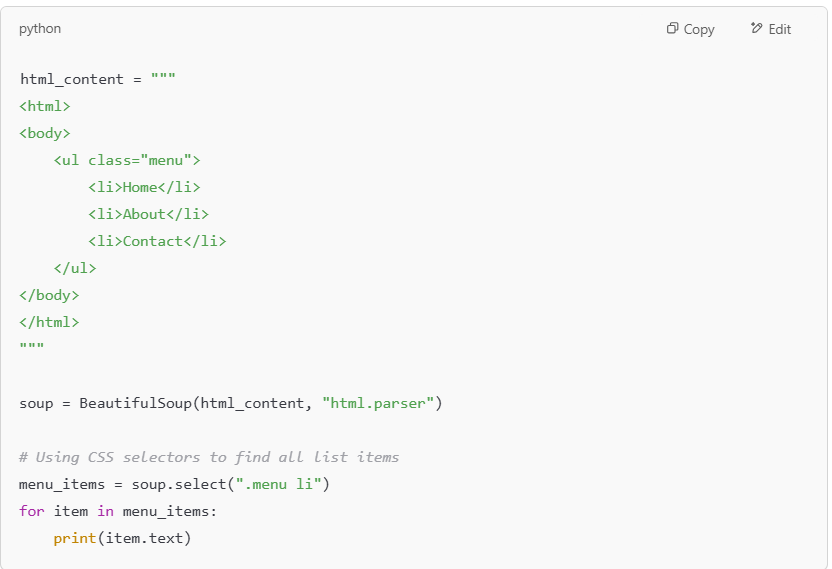
Output:
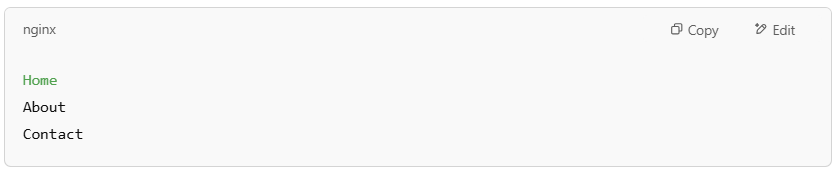
- .select(".menu li") finds all <li> tags inside an element with class menu.
6. Extracting Data from HTML Tables
If you're scraping structured web pages that include HTML tables, you can extract data row by row.
Example: Scraping an HTML Table
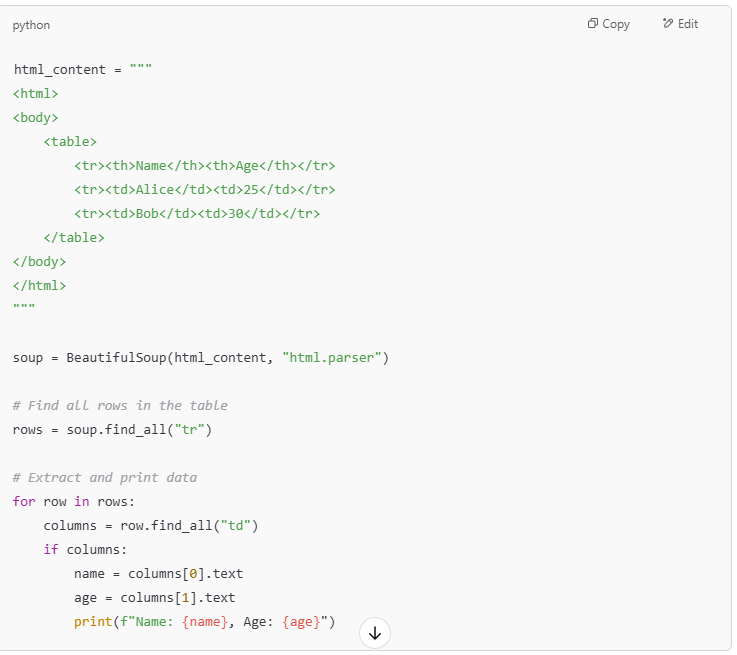
Output:

- .find_all("tr") gets all rows in the table.
- .find_all("td") extracts each cell’s text inside a row.
7. Extracting Data for a CSV File
If you’re scraping web pages and need to save data to a CSV file, use the csv module.
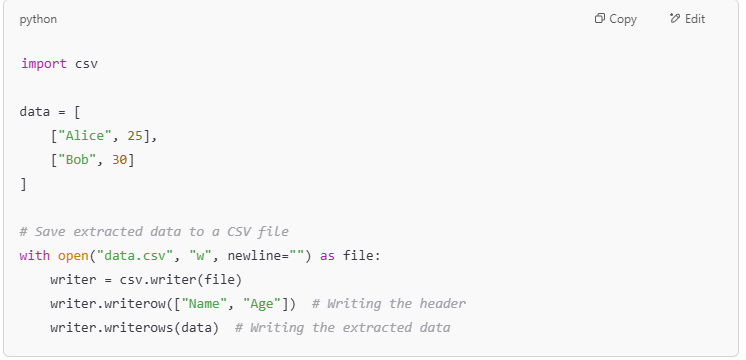
This code creates a CSV file named data.csv with extracted information.
Summary
- .find() retrieves the first occurrence of an element in an HTML document.
- .find_all() retrieves all occurrences of a specific HTML tag.
- Finding by class and ID helps locate targeted elements in an HTML file.
- CSS Selectors allow more advanced searching using .select().
- Parsing HTML tables lets you extract structured HTML data.
- Extracting data for a CSV file helps save scraped information for analysis.
With these techniques, you can extract data from various web pages, parse complex HTML structures, and organize information efficiently!
Navigating the HTML tree
Once you've extracted elements from an HTML file, you often need to navigate through its structure to access related content. BeautifulSoup makes this easy by treating the HTML document like a tree, where elements have parents, children, and siblings.
In this section, we'll explore how to move through this parse tree using .parent, .children, .next_sibling, and more.
Sample HTML Structure
Let’s start with a simple HTML document:
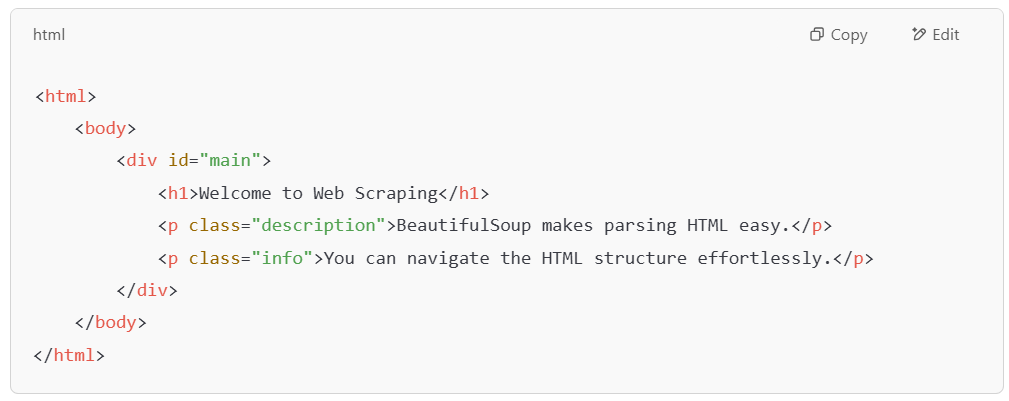
We will now use BeautifulSoup to navigate through this HTML structure step by step.
1. Accessing the Parent Element (.parent)
Every element in an HTML document has a parent. You can use .parent to move up the tree and find the element that contains the current tag.
Example: Finding the Parent of a Paragraph
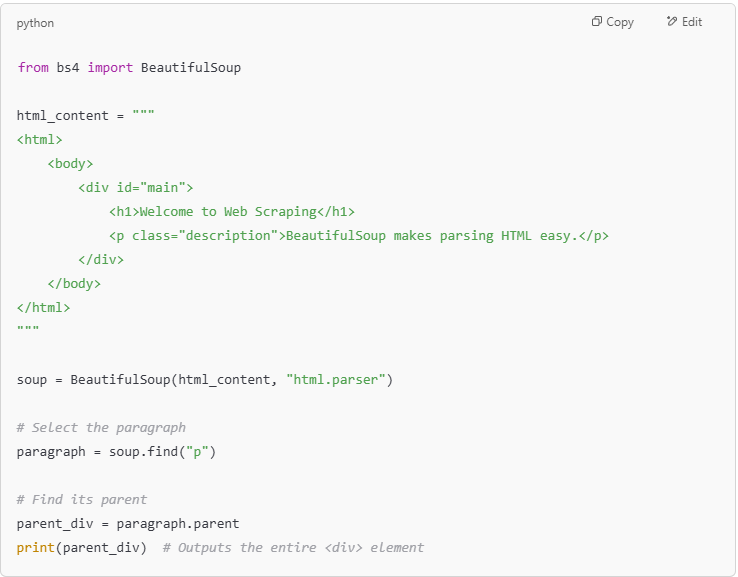
Explanation
- The <p> tag is inside the <div id="main"> tag.
- .parent moves up the tree to find the div that contains the paragraph.
2. Finding Child Elements (.children)
If an element contains multiple nested tags, you can access them using .children. This returns an iterator over all the direct children of a tag.
Example: Listing All Children of a <div>
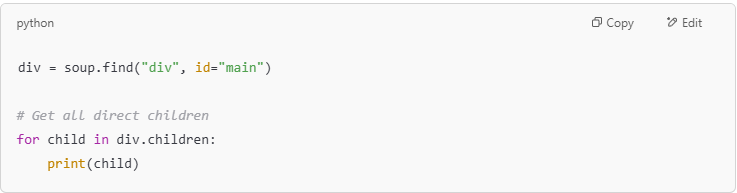
Output:

Explanation
- The <div> contains an <h1> and a <p>, so .children lists both.
- It only returns direct children, not deeper nested ones.
3. Accessing All Descendants (.descendants)
Unlike .children, which only finds direct children, .descendants finds all nested elements, even if they are deeper in the HTML structure.
Example: Listing All Descendants of <div>

Output:

Explanation
- .descendants retrieves both elements and text inside the <div>.
- It goes deeper than .children, capturing nested content.
4. Finding the Next and Previous Sibling Elements
In an HTML file, elements at the same level (inside the same parent) are siblings.
- .next_sibling finds the next element on the same level.
- .previous_sibling finds the previous element.
Example: Getting the Next Sibling

Explanation
- If the next sibling exists, it is returned.
- If there's whitespace between elements, .next_sibling might return a newline (\n).
- Use .next_sibling.next_sibling to skip over whitespace.
Example: Skipping Whitespace

Similarly, you can use .previous_sibling to get the preceding sibling.
5. Finding Parent of an Element (.find_parent())
The .find_parent() method works like .parent but allows searching up multiple levels.
Example: Finding a Parent with a Specific Tag

Explanation
- .find_parent("div") finds the closest parent <div>.
6. Finding All Parents of an Element (.find_parents())
If an element is deeply nested, you can get all parent elements using .find_parents().
Example: Listing All Parents

Output:

Explanation
- .find_parents() traces all parent elements up to <html>.
7. Finding Specific Parent Elements
Sometimes, you need to find the first matching parent of an element.
Example: Finding a Parent with a Specific ID

Explanation
- This will only return the first parent with id="main".
8. Navigating Using .next_element and .previous_element
- .next_element moves to the next tag or text inside the document, even if it’s deeply nested.
- .previous_element moves to the previous tag or text.
Example: Using .next_element

9. Using .find_next_sibling() and .find_previous_sibling()
If there are multiple sibling elements, use these methods to find specific ones.
Example: Finding the Next Paragraph

Output:

Example: Finding the Previous Sibling

Output:

Summary

Now you can navigate HTML files efficiently using BeautifulSoup. This helps extract structured data from web pages for web scraping projects.
- Image Requirements: Visual diagram of an HTML tree structure with arrows showing navigation.
Extracting text and attributes
When working with web scraping, one of the most common tasks is extracting specific pieces of HTML data - such as text content and attributes like href, src, class, and more. BeautifulSoup provides simple methods to extract this data efficiently.
In this section, we'll explore how to extract text from HTML tags using .text and .get_text(), extract attributes from HTML elements using .get() and .attrs, and work with real examples to extract links, images, and classes.
1. Extracting Text from HTML Elements
The .text and .get_text() methods allow you to retrieve the text content of an HTML tag, stripping out the actual markup.
Example: Extracting Text from a Paragraph
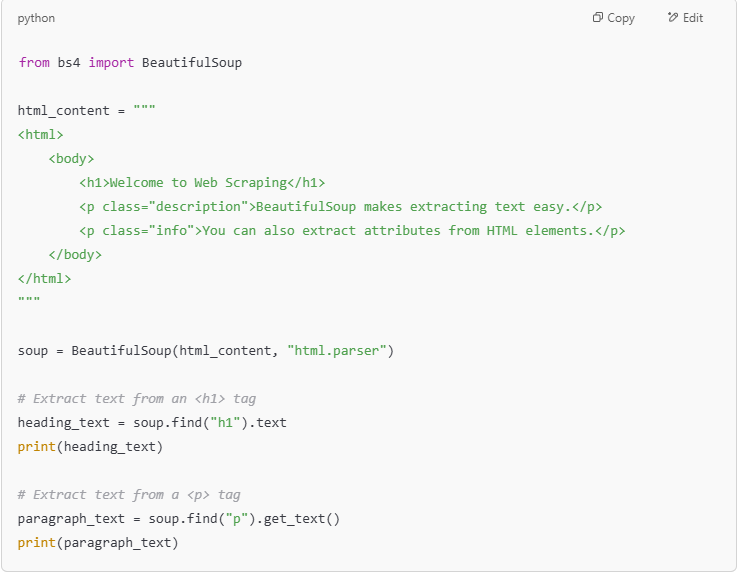
Output:

Explanation:
- .text and .get_text() return the text inside a tag, removing any HTML structure.
- Both methods work similarly, but .get_text() provides extra options (like stripping whitespace).
2. Extracting Attributes from HTML Elements
Besides text, HTML elements often contain attributes such as:
- href (links)
- src (images, videos)
- class (CSS styling)
- id (unique element identifiers)
To extract attributes, use:
- .get("attribute_name") – Returns the value of a specific attribute.
- .attrs["attribute_name"] – Another way to access attribute values.
Example: Extracting Links (href Attribute)
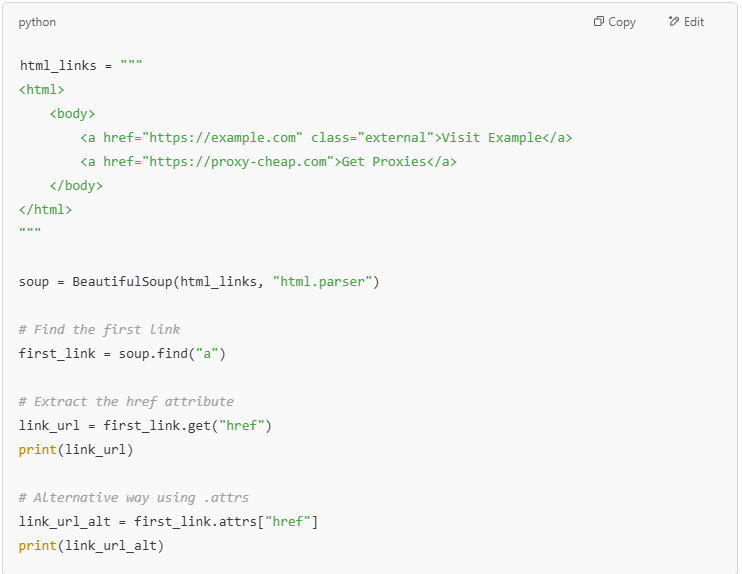
Output:

Explanation:
- .get("href") extracts the href (link URL).
- .attrs["href"] achieves the same result.
- If an attribute doesn't exist, .get() returns None, but .attrs[] will throw an error.
Use .get() when unsure if an attribute exists.
3. Extracting Image Sources (src Attribute)
Images are often stored inside <img> tags with a src attribute pointing to the image URL.
Example: Extracting an Image URL
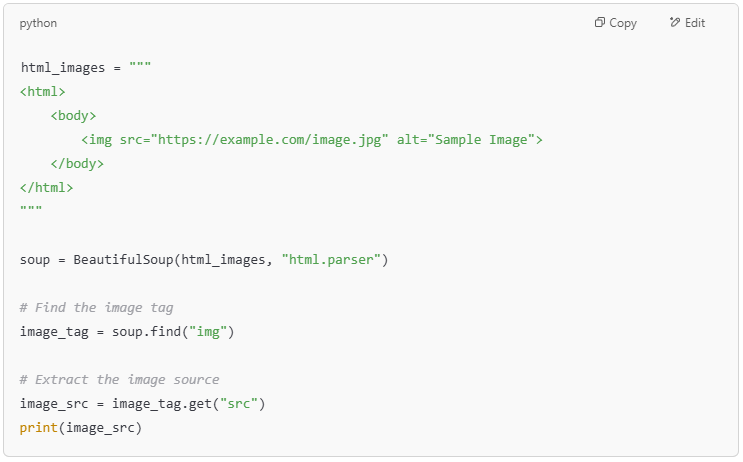
Output:

If an image URL is relative (e.g., /images/logo.png), combine it with the website's base URL for full access.
4. Extracting Multiple Attributes from an Element
You can retrieve all attributes of an element at once using .attrs.
Example: Extracting All Attributes from a Link

Output:

Notice: The class attribute returns a list, as elements can have multiple classes.
5. Extracting CSS Classes from Elements
Since an element can have multiple CSS classes, they are returned as a list.
Example: Extracting Class Names

Output:
Tip: To check if an element has a specific class, use:

6. Extracting Table Data (td, th)
Extracting data from HTML tables is useful for data extraction projects.
Example: Extracting Table Data
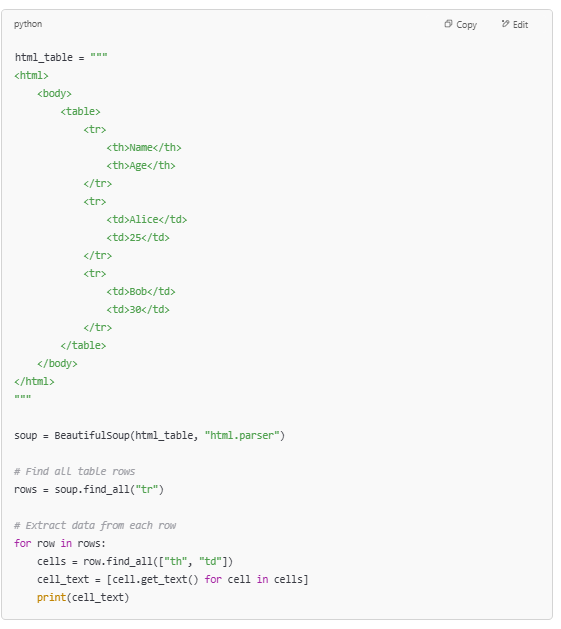
Output:

Tip: You can store extracted data in a CSV file for later analysis.
Summary

Common use cases for BeautifulSoup
BeautifulSoup is widely used in web scraping to extract valuable web data from HTML documents and XML files. Whether you need to collect article titles, product listings, or extract links from a website, BeautifulSoup makes the process simple and efficient. However, scraping websites can sometimes lead to IP bans. Using residential proxies from Proxy-Cheap can help you avoid detection and bypass IP blocks when scraping at scale.
Let’s explore some of the most common BeautifulSoup use cases:
1. Extracting Article Titles from a Blog
News websites, blogs, and online magazines use structured HTML to display article titles and summaries. You can use BeautifulSoup to extract all headlines from a webpage.
Example: Scraping Article Titles

Output:

Key Takeaways:
- The .find_all() method helps extract all article titles.
- We use CSS selectors (class_="article-title") to locate elements.
- This method is useful for news aggregation, research, or content monitoring.
3. Scraping Product Listings from an E-Commerce Website
E-commerce platforms use HTML tables and structured data to display products. BeautifulSoup helps scrape product names, prices, and links from online stores.
Example: Extracting Product Listings

Output:

Why This is Useful?
- Helps monitor product prices for price tracking tools.
- Useful for competitor analysis in e-commerce.
- Enables building a custom product catalog.
3. Extracting All Links from a Web Page
Web pages contain multiple links (<a> tags) pointing to other resources. You can use BeautifulSoup to collect all URLs from a page.
Example: Extracting All Hyperlinks
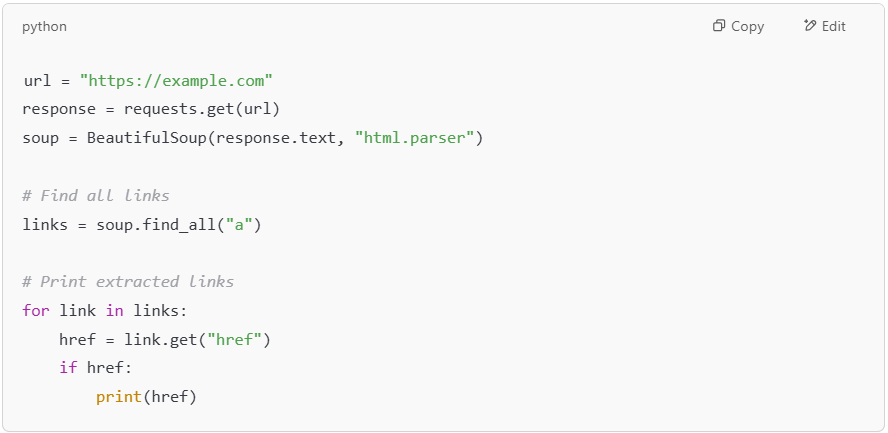
Output:

Tip: If the link is relative (e.g., /about), prepend the website’s base URL for full access.
4. Scraping Image URLs from a Web Page
Image data is essential in e-commerce, media analysis, and machine learning. You can extract image URLs from <img> tags easily.
Example: Extracting Image Sources
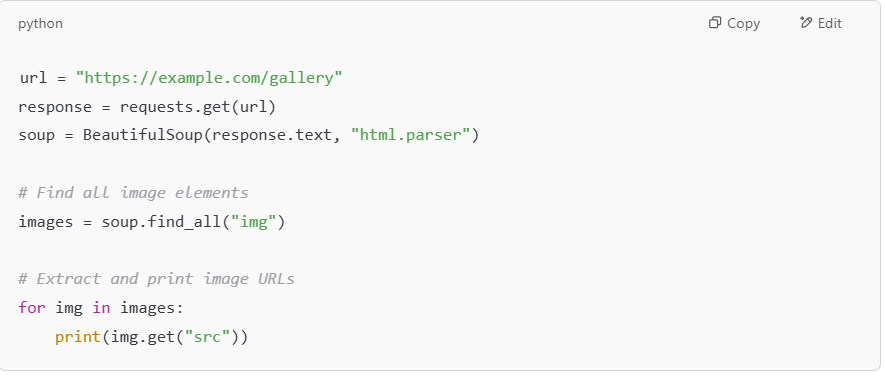
Output:

Tip: If an image URL is relative (e.g., /images/pic.jpg), prepend the domain name.
5. Scraping Contact Information (Emails & Phone Numbers)
Extracting emails and phone numbers from a website is useful for lead generation and business intelligence.
Example: Extracting Emails & Phone Numbers
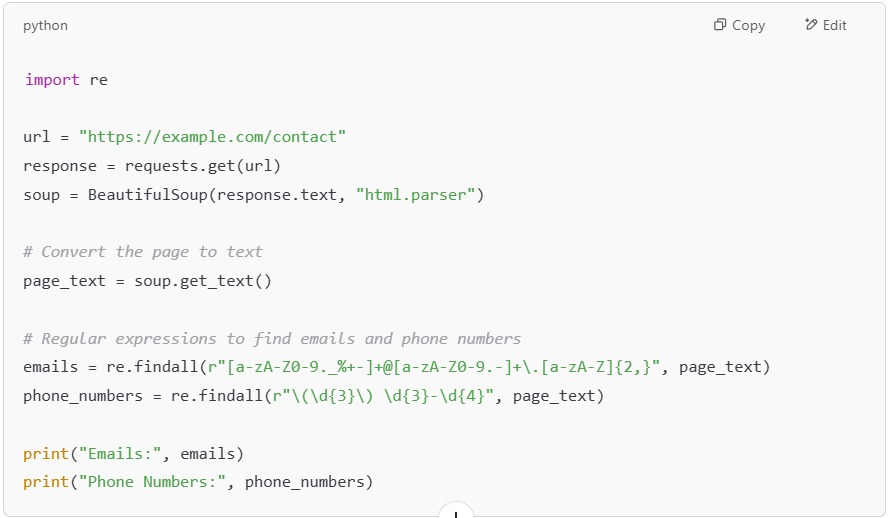
Output:

Note: Many sites hide emails behind JavaScript, so this approach works best for static pages.
Using Proxies to Avoid Blocks
Websites often block scrapers if too many requests come from the same IP. To prevent this, use residential proxies from Proxy-Cheap to rotate your IP address.
Example: Using a Proxy with Requests

Why Use a Proxy?
- Avoids IP bans when scraping frequently.
- Bypasses regionally-restricted content.
- Ensures stealth scraping for large-scale projects.
BeautifulSoup is a powerful tool for parsing HTML data and extracting useful information from web pages. Whether you're scraping blog titles, product listings, images, or emails, BeautifulSoup simplifies the process.
However, scraping large websites requires caution. Using residential proxies from Proxy-Cheap helps avoid IP bans and ensures seamless data extraction.
Combining BeautifulSoup with requests
When using BeautifulSoup for web scraping, you need a way to fetch live webpage content from the internet. This is where the requests library comes in. The requests module allows us to send HTTP requests to a website, retrieve its HTML document, and pass it to BeautifulSoup for parsing.
Why Combine BeautifulSoup with requests?
- Retrieve live data from websites.
- Parse web data dynamically instead of using saved HTML files.
- Extract data from real-time sources like blogs, product pages, and stock market sites.
1. Installing requests and BeautifulSoup
Before we start, ensure both requests and BeautifulSoup are installed. You can install them using pip:

Now, you are ready to fetch web pages and parse HTML data.
2. Fetching a Web Page Using requests
Let's start by fetching a webpage using the requests module and passing its HTML document to BeautifulSoup.
Example: Fetching and Parsing HTML

Explanation:
- requests.get(url) → Sends a request to fetch the HTML file of the website.
- soup = BeautifulSoup(response.text, "html.parser") → Converts the HTML data into a BeautifulSoup object.
- soup.title.text → Extracts the page title from the HTML.
Output:

3. Using Headers to Mimic a Browser
Many websites block automated scrapers by detecting requests that don’t have a browser User-Agent. To avoid this, we can send custom headers that make our request look like it’s coming from a real web browser.
Example: Sending Headers in a Request

- headers → This dictionary mimics a real browser request.
- User-Agent → Prevents the website from blocking your request.
4. Handling HTTP Errors (404, 403, etc.)
Websites may return errors like 404 (Not Found) or 403 (Forbidden). To avoid crashing your script, you should check the response status before parsing the HTML.
Example: Handling HTTP Errors

- response.status_code → Checks if the request was successful.
- If the page is not available, the script won’t break.
5. Extracting Data from a Live Web Page
Let’s fetch a real website and extract all the article titles.
Example: Extracting Article Titles

- find_all("h2", class_="article-title") → Extracts all article titles.
- Live data scraping instead of using a saved HTML document.
Output:

6. Using Proxies to Avoid Blocks
If a website blocks your requests, you can use a proxy to change your IP address. Services like Proxy-Cheap provide residential proxies that help you scrape without getting blocked.
Example: Using a Proxy with requests
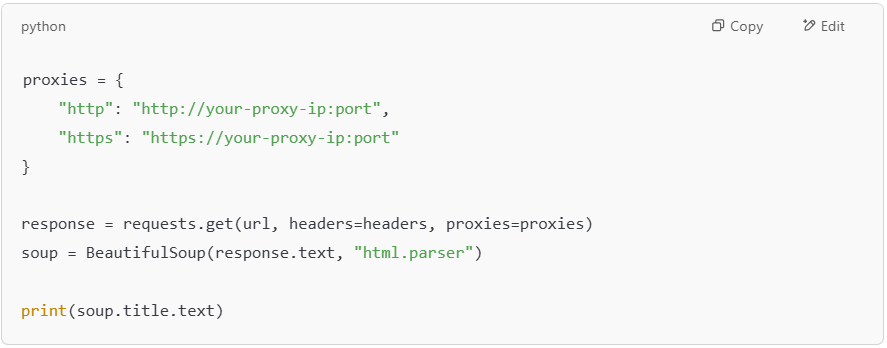
- proxies → Routes the request through a proxy server.
- Helps bypass IP bans and access regionally limited content.
Combining requests with BeautifulSoup is a powerful way to scrape live web pages. By adding headers, handling errors, and using proxies, you can scrape more efficiently while avoiding blocks.
- Image Requirements: Screenshot of code showing the full process: requests.get() + parsing HTML.
Tips to avoid common errors
When using BeautifulSoup for web scraping, beginners often run into errors that can break their scripts. These errors usually stem from using the wrong parser, dealing with broken HTML, or missing elements in the HTML document.
Here’s a detailed guide on common mistakes and how to fix them.
1. Choosing the Right HTML Parser
Common Issue:
You may encounter an error like this when trying to parse an HTML file:

This happens when BeautifulSoup doesn't know which parser to use.
Fix:
Make sure you install lxml or use Python’s built-in html.parser.

Specify the parser when creating the BeautifulSoup object:
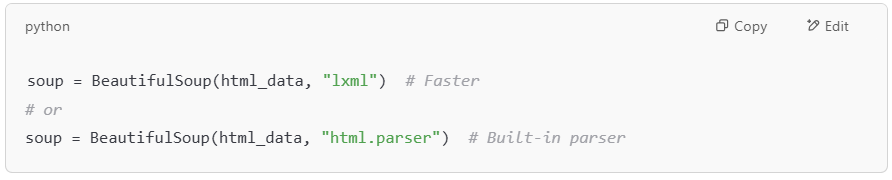
2. Handling Broken or Incomplete HTML
Common Issue:
Some web pages contain poorly formatted HTML with missing html tags. This can cause BeautifulSoup to fail when trying to extract data.
Fix:
- BeautifulSoup automatically fixes broken HTML, but you can also validate the HTML document before parsing using an online validator like W3C Validator.
- Use try-except blocks to handle errors gracefully.
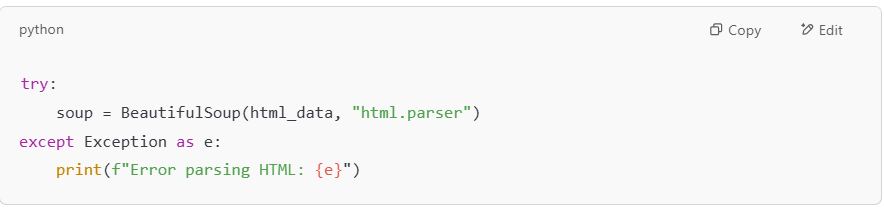
3. Finding Non-Existent Elements
Common Issue:
You may get NoneType errors when trying to access an HTML tag that doesn’t exist in the HTML document:

This happens when BeautifulSoup can’t find the requested HTML elements.
Fix:
Use .get() or check if the element exists before extracting html data.

4. Handling Dynamic Web Pages
Common Issue:
Some web pages load content using JavaScript instead of static HTML. BeautifulSoup cannot parse web data that is loaded dynamically.
Fix:
- Use Selenium or Scrapy for JavaScript-rendered pages.
- Check if the web scraping API you're using provides an option for rendering JavaScript.
Example using Selenium:
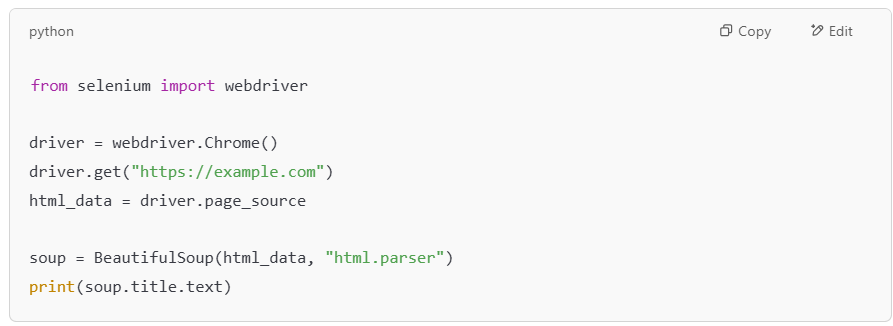
5. Handling HTTP Errors (403, 404, etc.)
Common Issue:
Some websites block requests and return errors like 403 Forbidden.
Fix:
- Use headers to mimic a real browser request:
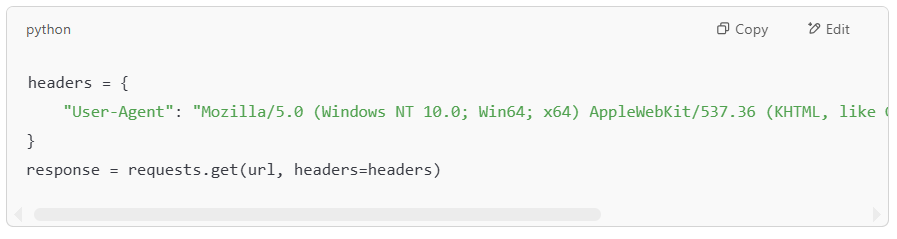
- Use proxies to rotate your IP address. Services like Proxy-Cheap can help avoid IP bans.
6. Extracting Data from Tables and Lists
Common Issue:
Scraping HTML tables can be tricky if you don’t use the right method.
Fix:
- Use .find_all("tr") to extract rows from html tables:
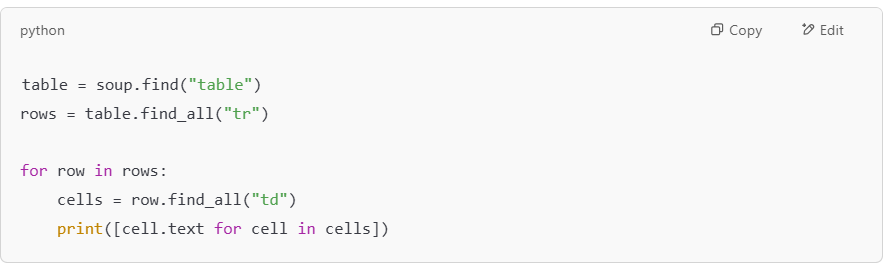
- Export data to a CSV file for easy use:
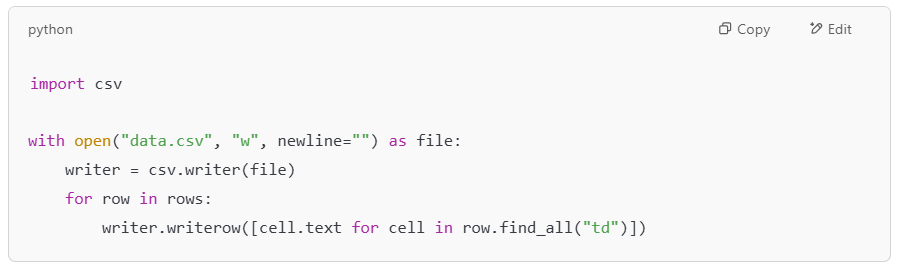
7. Avoiding IP Bans
Common Issue:
If you send too many requests, websites may block your IP.
Fix:
Use time delays between requests:

- Rotate IPs using Proxy-Cheap’s residential proxies:

Common BeautifulSoup Errors – FAQ
Q1: My script runs but returns an empty result. Why?
The website may be using JavaScript to load content. Use Selenium instead.
Q2: I get AttributeError: 'NoneType' object has no attribute 'text'. What should I do?
The element is missing. Use if statements before accessing .text.
Q3: Why do I get 403 Forbidden when scraping?
The site is blocking bots. Try adding a User-Agent or using a proxy.
Q4: My script works on some pages but not others. What’s wrong?
The HTML structure might be different on each page. Use .prettify() to inspect the HTML document.
Q5: How can I scrape multiple pages automatically?
Use a loop with a paginated URL:
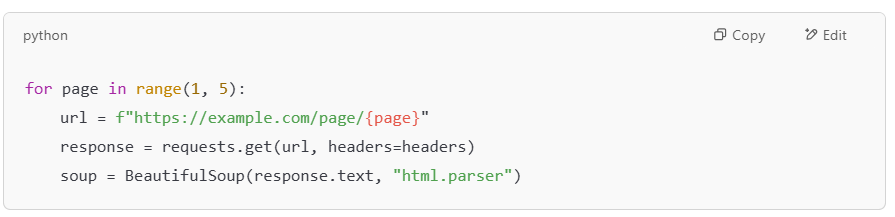
By following these troubleshooting tips, you can avoid the most common BeautifulSoup errors and make your web scraping projects run smoothly.
Conclusion
BeautifulSoup is an incredibly powerful and beginner-friendly Python library that simplifies data parsing from web pages. Whether you are extracting HTML elements, locating specific print tags, or navigating through an HTML structure, BeautifulSoup makes the process intuitive and efficient. It allows you to access and manipulate content from a target website with just a few lines of code, making it a go-to tool for anyone interested in web scraping.
By using BeautifulSoup, you can parse HTML with ease, even when dealing with messy or inconsistent data. It provides multiple methods to find elements, navigate through the HTML tree, and extract key details like text content, attributes, and links. With additional tools like the requests library, you can fetch live web pages, process their data, and store valuable insights for further analysis.
Now that you have a solid understanding of how BeautifulSoup works, it's time to take the next steps! Start by practicing on a simple HTML document, experimenting with different find() and find_all() methods, and testing your scripts on a target website. Once comfortable, consider exploring more advanced scraping techniques like handling dynamic pages, using proxies, or integrating with APIs. Additionally, you can save extracted data into structured formats like a CSV file for further analysis.
Web scraping is an exciting skill with endless possibilities. Whether you want to gather news articles, analyze product listings, or extract research data, BeautifulSoup provides the foundation to get started. So, go ahead - pick a website, write a script, and start exploring the world of data parsing with BeautifulSoup!